Как сложить и вычесть две матрицы в C ++, Python и JavaScript
Матрица – это прямоугольный массив чисел, символов или выражений, расположенных в строках и столбцах. Эта прямоугольная сетка чисел обычно используется в математике, электротехнике и информатике. Матрицы изначально создавались для описания системы линейных уравнений.
Сейчас матрицы широко используются в обработке изображений, генетическом анализе, больших данных и программировании. Сложение и вычитание матриц – две наиболее распространенные операции с матрицами. В этой статье вы узнаете, как складывать и вычитать две матрицы.
Правила сложения матриц
Следуйте этим правилам, чтобы добавить две матрицы:
- Две матрицы могут быть добавлены только в том случае, если они одного порядка.
- Если две матрицы имеют одинаковый порядок, добавьте соответствующие элементы двух матриц, т. Е. Добавьте элементы, которые имеют одинаковые позиции.
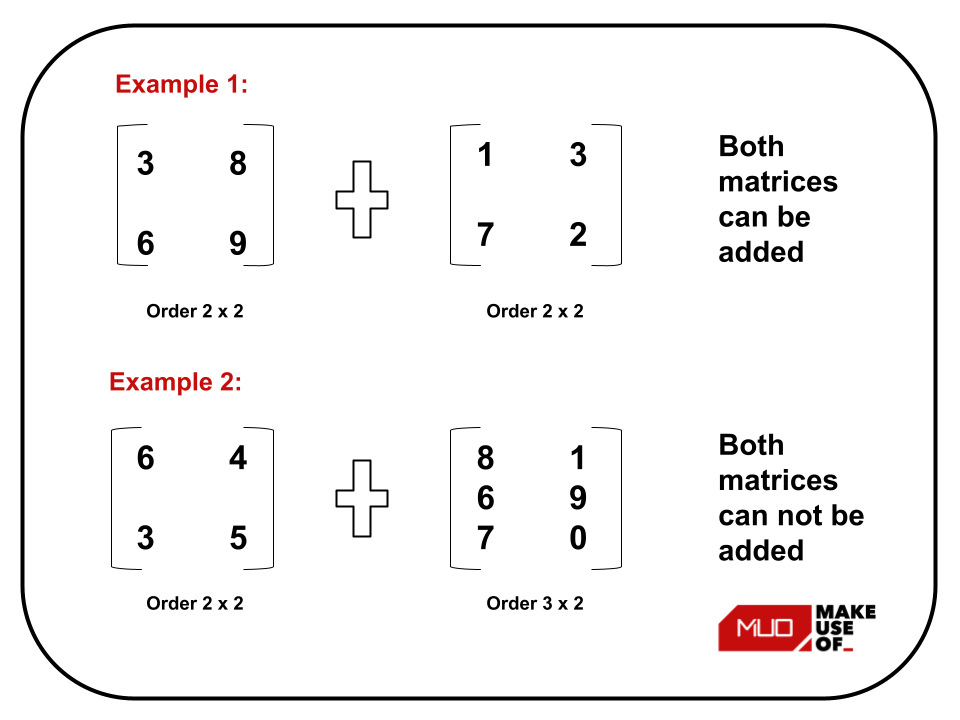
В примере 1 матрицы могут быть добавлены, потому что они имеют одинаковый порядок. В примере 2 матрицы не могут быть добавлены, потому что они имеют разный порядок.
Программа на C ++ для добавления двух матриц
Ниже представлена программа на C ++ для добавления двух матриц:
// C++ program for addition of two matrices
#include <bits/stdc++.h>
using namespace std;
// The order of the matrix is 3 x 3
#define size1 3
#define size2 3
// Function to add matrices mat1[][] & mat2[][],
// and store the result in matrix result[][]
void addMatrices(int mat1[][size2], int mat2[][size2], int result[][size2])
{
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
result[i][j] = mat1[i][j] + mat2[i][j];
}
}
}
// Driver code
int main()
{
// 1st Matrix
int mat1[size1][size2] = { {9, 8, 7},
{6, 8, 0},
{5, 9, 2} };
// 2nd Matrix
int mat2[size1][size2] = { {4, 7, 6},
{8, 8, 2},
{7, 3, 5} };
// Matrix to store the result
int result[size1][size2];
// Calling the addMatrices() function
addMatrices(mat1, mat2, result);
cout << "mat1 + mat2 = " << endl;
// Printing the sum of the 2 matrices
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
cout << result[i][j] << " ";
}
cout << endl;
}
return 0;
}
Выход:
mat1 + mat2 =
13 15 13
14 16 2
12 12 7
Программа Python для добавления двух матриц
Ниже представлена программа Python для добавления двух матриц:
# Python program for addition of two matrices
# The order of the matrix is 3 x 3
size1 = 3
size2 = 3
# Function to add matrices mat1[][] & mat2[][],
# and store the result in matrix result[][]
def addMatrices(mat1,mat2,result):
for i in range(size1):
for j in range(size2):
result[i][j] = mat1[i][j] + mat2[i][j]
# driver code
# 1st Matrix
mat1 = [ [9, 8, 7],
[6, 8, 0],
[5, 9, 2] ]
# 2nd Matrix
mat2 = [ [4, 7, 6],
[8, 8, 2],
[7, 3, 5] ]
# Matrix to store the result
result = mat1[:][:]
# Calling the addMatrices function
addMatrices(mat1, mat2, result)
# Printing the sum of the 2 matrices
print("mat1 + mat2 = ")
for i in range(size1):
for j in range(size2):
print(result[i][j], " ", end='')
print()
Выход:
mat1 + mat2 =
13 15 13
14 16 2
12 12 7
Программа на C для добавления двух матриц
Ниже представлена программа на языке C для добавления двух матриц:
// C program for addition of two matrices
#include <stdio.h>
// The order of the matrix is 3 x 3
#define size1 3
#define size2 3
// Function to add matrices mat1[][] & mat2[][],
// and store the result in matrix result[][]
void addMatrices(int mat1[][size2], int mat2[][size2], int result[][size2])
{
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
result[i][j] = mat1[i][j] + mat2[i][j];
}
}
}
// Driver code
int main()
{
// 1st Matrix
int mat1[size1][size2] = { {9, 8, 7},
{6, 8, 0},
{5, 9, 2} };
// 2nd Matrix
int mat2[size1][size2] = { {4, 7, 6},
{8, 8, 2},
{7, 3, 5} };
// Matrix to store the result
int result[size1][size2];
// Calling the addMatrices function
addMatrices(mat1, mat2, result);
printf("mat1 + mat2 = n");
// Printing the sum of the 2 matrices
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
printf("%d ", result[i][j]);
}
printf("n");
}
return 0;
}
Выход:
mat1 + mat2 =
13 15 13
14 16 2
12 12 7
Программа на JavaScript для добавления двух матриц
Ниже приведена программа на JavaScript для добавления двух матриц:
<script>
// JavaScript program for addition of two matrices
// The order of the matrix is 3 x 3
let size1 = 3;
let size2 = 3;
// Function to add matrices mat1[][] & mat2[][],
// and store the result in matrix result[][]
function addMatrices(mat1, mat2, result) {
for (let i = 0; i < size1; i++) {
for (let j = 0; j < size2; j++) {
result[i][j] = mat1[i][j] + mat2[i][j];
}
}
}
// Driver code
// 1st Matrix
mat1 = [ [9, 8, 7],
[6, 8, 0],
[5, 9, 2] ]
// 2nd Matrix
mat2 = [ [4, 7, 6],
[8, 8, 2],
[7, 3, 5] ]
// Matrix to store the result
let result = new Array(size1);
for (let k = 0; k < size1; k++) {
result[k] = new Array(size2);
}
// Calling the addMatrices function
addMatrices(mat1, mat2, result);
document.write("mat1 + mat2 = <br>")
// Printing the sum of the 2 matrices
for (let i = 0; i < size1; i++) {
for (let j = 0; j < size2; j++) {
document.write(result[i][j] + " ");
}
document.write("<br>");
}
</script>
Выход:
mat1 + mat2 =
13 15 13
14 16 2
12 12 7
Правила вычитания матриц
Следуйте этим правилам, чтобы вычесть две матрицы:
- Две матрицы можно вычесть, только если они одного порядка.
- Если две матрицы имеют одинаковый порядок, вычтите соответствующие элементы из двух матриц, то есть вычтите элементы, которые имеют одинаковые позиции.
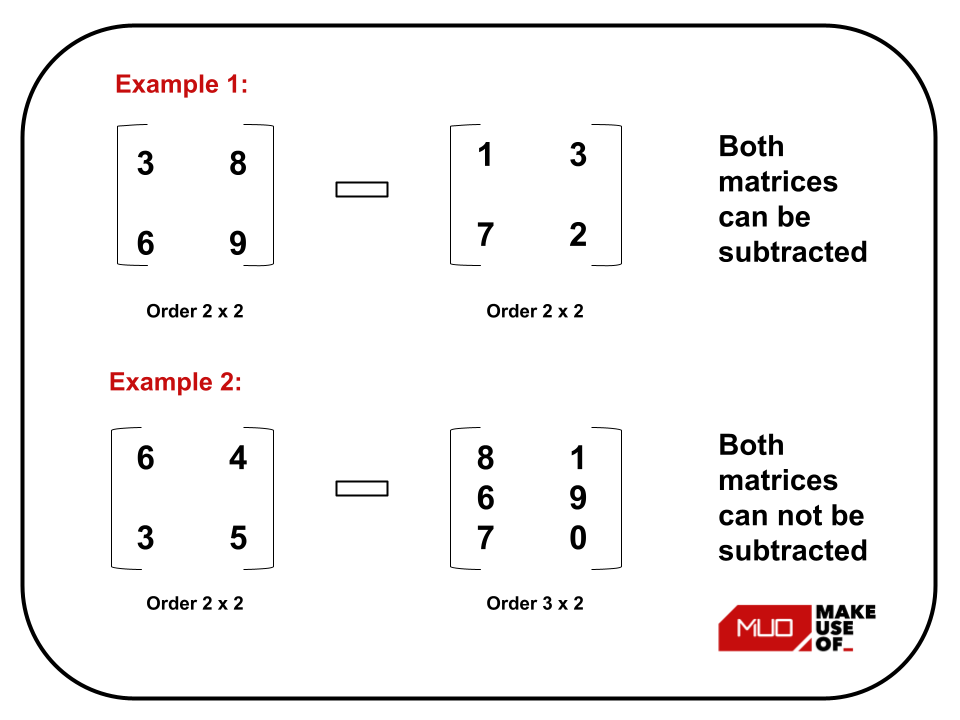
В примере 1 матрицы могут быть вычтены, потому что они имеют одинаковый порядок. В примере 2 нельзя вычесть матрицы, потому что они имеют разный порядок.
Программа C ++ для вычитания двух матриц
Ниже представлена программа на C ++ для вычитания двух матриц:
// C++ program for subtraction of two matrices
#include <bits/stdc++.h>
using namespace std;
// The order of the matrix is 3 x 3
#define size1 3
#define size2 3
// Function to subtract matrices mat1[][] & mat2[][],
// and store the result in matrix result[][]
void subtractMatrices(int mat1[][size2], int mat2[][size2], int result[][size2])
{
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
result[i][j] = mat1[i][j] - mat2[i][j];
}
}
}
// Driver code
int main()
{
// 1st Matrix
int mat1[size1][size2] = { {9, 8, 7},
{6, 8, 0},
{5, 9, 2} };
// 2nd Matrix
int mat2[size1][size2] = { {4, 7, 6},
{8, 8, 2},
{7, 3, 5} };
// Matrix to store the result
int result[size1][size2];
// Calling the subtractMatrices() function
subtractMatrices(mat1, mat2, result);
cout << "mat1 - mat2 = " << endl;
// Printing the difference of the 2 matrices (mat1 - mat2)
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
cout << result[i][j] << " ";
}
cout << endl;
}
return 0;
}
Выход:
mat1 - mat2 =
5 1 1
-2 0 -2
-2 6 -3
Программа Python для вычитания двух матриц
Ниже представлена программа Python для вычитания двух матриц:
# Python program for subtraction of two matrices
# The order of the matrix is 3 x 3
size1 = 3
size2 = 3
# Function to subtract matrices mat1[][] & mat2[][],
# and store the result in matrix result[][]
def subtractMatrices(mat1,mat2,result):
for i in range(size1):
for j in range(size2):
result[i][j] = mat1[i][j] - mat2[i][j]
# driver code
# 1st Matrix
mat1 = [ [9, 8, 7],
[6, 8, 0],
[5, 9, 2] ]
# 2nd Matrix
mat2 = [ [4, 7, 6],
[8, 8, 2],
[7, 3, 5] ]
# Matrix to store the result
result = mat1[:][:]
# Calling the subtractMatrices function
subtractMatrices(mat1, mat2, result)
# Printing the difference of the 2 matrices (mat1 - mat2)
print("mat1 - mat2 = ")
for i in range(size1):
for j in range(size2):
print(result[i][j], " ", end='')
print()
Выход:
mat1 - mat2 =
5 1 1
-2 0 -2
-2 6 -3
Программа на C для вычитания двух матриц
Ниже представлена программа на языке C для вычитания двух матриц:
// C program for subtraction of two matrices
#include <stdio.h>
// The order of the matrix is 3 x 3
#define size1 3
#define size2 3
// Function to subtract matrices mat1[][] & mat2[][],
// and store the result in matrix result[][]
void subtractMatrices(int mat1[][size2], int mat2[][size2], int result[][size2])
{
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
result[i][j] = mat1[i][j] - mat2[i][j];
}
}
}
// Driver code
int main()
{
// 1st Matrix
int mat1[size1][size2] = { {9, 8, 7},
{6, 8, 0},
{5, 9, 2} };
// 2nd Matrix
int mat2[size1][size2] = { {4, 7, 6},
{8, 8, 2},
{7, 3, 5} };
// Matrix to store the result
int result[size1][size2];
// Calling the subtractMatrices() function
subtractMatrices(mat1, mat2, result);
printf("mat1 - mat2 = n");
// Printing the difference of the 2 matrices (mat1 - mat2)
for (int i = 0; i < size1; i++)
{
for (int j = 0; j < size2; j++)
{
printf("%d ", result[i][j]);
}
printf("n");
}
return 0;
}
Выход:
mat1 - mat2 =
5 1 1
-2 0 -2
-2 6 -3
Программа на JavaScript для вычитания двух матриц
Ниже приведена программа на JavaScript для вычитания двух матриц:
<script>
// JavaScript program for subtraction of two matrices
// The order of the matrix is 3 x 3
let size1 = 3;
let size2 = 3;
// Function to subtract matrices mat1[][] & mat2[][],
// and store the result in matrix result[][]
function subtractMatrices(mat1, mat2, result) {
for (let i = 0; i < size1; i++) {
for (let j = 0; j < size2; j++) {
result[i][j] = mat1[i][j] - mat2[i][j];
}
}
}
// Driver code
// 1st Matrix
mat1 = [ [9, 8, 7],
[6, 8, 0],
[5, 9, 2] ]
// 2nd Matrix
mat2 = [ [4, 7, 6],
[8, 8, 2],
[7, 3, 5]]
// Matrix to store the result
let result = new Array(size1);
for (let k = 0; k < size1; k++) {
result[k] = new Array(size2);
}
// Calling the subtractMatrices function
subtractMatrices(mat1, mat2, result);
document.write("mat1 - mat2 = <br>")
// Printing the difference of the 2 matrices (mat1 - mat2)
for (let i = 0; i < size1; i++) {
for (let j = 0; j < size2; j++) {
document.write(result[i][j] + " ");
}
document.write("<br>");
}
</script>
Выход:
mat1 - mat2 =
5 1 1
-2 0 -2
-2 6 -3
Если вы хотите взглянуть на полный исходный код, использованный в этой статье, вот репозиторий GitHub .
Расширьте свои возможности программирования
Вы можете расширить свои возможности программирования, практикуя различные задачи программирования. Решение этих проблем программирования помогает вам разработать базовые принципы программирования. Это необходимо знать, если вы хотите стать эффективным программистом.